Below is close to what I'm using in my CarPlay SceneDelegate. I'm still seeing the same results as before and I've minimized most of the code to reduce any chance of more issues arising. When the knob is focused on the tab selection I'm still getting a flicker upon calling func updateSections(_ sections: [CPListSection])
. When an item is selected in the list and my app refreshes the list of items, then selected highlighted cell jumps from index 1 to random indexes in the list. My app handles a notification and refreshes the list template section and without updating sections the list never updates when adding or removing items.
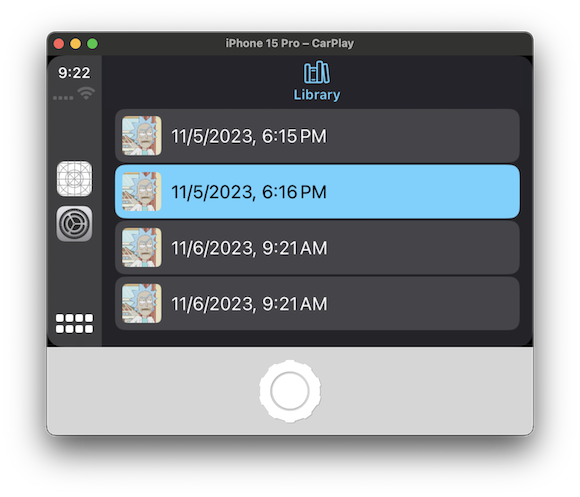
class MyCarPlaySceneDelegate: NSObject, CPTemplateApplicationSceneDelegate, CPInterfaceControllerDelegate {
private var interfaceController: CPInterfaceController?
private var template: CPListTemplate?
private var items: [Item] = [] /// Maintain the list of items
var tabTemplates = [CPTemplate]()
func listTemplate() -> CPListTemplate {
loadItems() /// Load items here to avoid code duplication
var list: [CPListItem] = []
for item in items {
list.append(item)
}
let template = CPListTemplate(title: "Library", sections: [CPListSection(items: list)])
template.updateSections([CPListSection(items: list)]) /// Without this here the second value in the list is highlighted
self.template = template
return template
}
func loadItems() {
/// Loading data here
}
/// handle changes here to reload list
@objc func handleNotification(_ notification: Notification) {
var list: [CPListItem] = []
if let template = self.template {
loadItems() /// Reload items when a notification is received
template.updateSections([CPListSection(items: list)])
}
}
func templateApplicationScene(_ templateApplicationScene: CPTemplateApplicationScene, didConnect interfaceController: CPInterfaceController) {
NotificationCenter.default.addObserver(
self,
selector: #selector(handleNotification(_:)),
name: Notification.Name.HELLOWORLD,
object: nil
)
self.interfaceController = interfaceController
self.interfaceController?.delegate = self
let template = listTemplate()
tabTemplates.append(template)
interfaceController.setRootTemplate(CPTabBarTemplate(templates: tabTemplates), animated: true, completion: nil)
}
func templateApplicationScene(_ templateApplicationScene: CPTemplateApplicationScene, didDisconnectInterfaceController interfaceController: CPInterfaceController) {
self.interfaceController = nil
NotificationCenter.default.removeObserver(self)
}
}