Retired Document
Important: This document may not represent best practices for current development. Links to downloads and other resources may no longer be valid.
Printing for Carbon Applications
Printing under Mac OS X and Carbon is very similar to printing under Win32. In addition to having separate setup tasks before the printing begins and cleanup tasks after it has finished, the main print loop for both platforms is essentially the same:
Declare the beginning of the print job (
StartDoc
for Win32,PMSessionBeginDocument
for Mac OS X).Declare the beginning of a page (
StartPage
for Win32,PMSessionBeginPage
for Mac OS X).Issue (largely) the same drawing commands as you would to the screen, "drawing" instead to an image area meant for a printer.
Declare the end of a page (
EndPage
for Win32,PMSessionEndPage
for Mac OS X).Declare the end of the print job (
EndDoc
for Win32,PMSessionEndDocument
for Mac OS X).
In addition, you must create routines that handle all the errors that might occur during printing.
The Printing User Interface
The three dialogs and windows that are associated with Mac OS X printing are not that different in function from their Windows counterparts. These are the Print dialog, the Page Setup dialog, and the Print Center windows.
The first, the Print dialog, enables the user to set the various parameters associated with the document about to be printed. This dialog can display different panes depending on which menu item user selects from the Features pop-up menu. Some panes are standard to all Print dialogs, while others are specific to the printer or the application. You can add your own panes by implementing them as printing dialog extensions (PDEs); see the Extending Printing Dialogs and Printing Plug-In Interfaces Reference books for details.
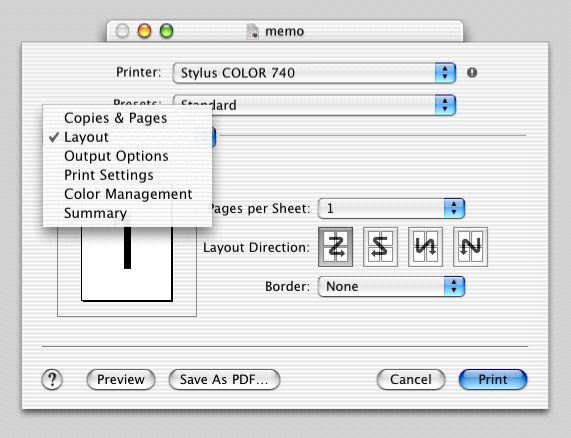
Users invoke the Page Setup dialog only if they want to change the default values of certain formatting properties. As with the Print dialog, you can add application-specific panes to the Page Setup dialog.
Print Center is a utility supplied with Mac OS X; its function is to enable users to view and manipulate printers and their print jobs. This utility displays a single window named Printer List (see below). Users can see a printer's current print jobs and can suspend them, delete them, or change the order in which they print.
Carbon Printing Manager
The Carbon Printing Manager is the API you should use to implement printing when you port your Win32 application to Mac OS X, and the book Supporting Printing in Your Carbon Application is the best starting place for understanding how to do so. The subsections that follow give an overview of the actions your application must take to prepare for and perform printing. (Names in parentheses are the names of Carbon Printing Manager routines that you will use.) You will see that the process is similar in structure to that of printing in a procedural Win32 application.
Setting up the Page Format
When the user executes the Page Setup menu item, your application should do the following:
Create a printing session object (
PMCreateSession
).Obtain a valid page format object for the document (custom routine from your application).
Specify that the Page Setup dialog should display itself as a sheet (
PMSessionUseSheets
).Display the Page Setup dialog (
PMSessionPageSetupDialog
).Save the values from this dialog for future use.
Release the printing session object (
PMRelease
) and handle any errors.
Executing the Print Command
When the user executes the Print menu item, your application should do the following:
Create a printing session object (
PMCreateSession
).Create a a valid page format object (
PMCreatePageFormat
), if one doesn't exist.Create a print settings object (
PMCreatePrintSettings)
.Display the Print dialog. (Here, the user clicks either the Print button or the Cancel button, and the appropriate actions occur.)
Release the printing session object (
PMRelease
), set the print settings object toNULL
, and handle any errors.
When the user clicks the Print button, your application should execute its print-loop code.
The Print Loop
Your print-loop code should do the following:
Determine the maximum number of pages that can possibly be printed, then give that information to the computer.
Calculate the page numbers of the first and last pages to be printed using data from the Print dialog (
PMGetFirstPage
,PMGetLastPage
), then give that information to the computer.Create a new print job (
PMSessionBeginDocument
).Set up a loop for drawing each page in the specified range. (Steps 5 through 12 are performed for each page.)
Tell the printing system that the code that follows begins a new page (
PMSessionBeginPage
).Save the current graphics port (
GetPort
).Get the graphics printing port for the page to be printed (
PMSessionGetGraphicsContext
).Set that graphics port to be the current QuickDraw graphics port (
SetPort
).Get the rectangle that defines the area in which drawing can occur (
GetPortBounds
).Call the code that draws the current page.
Restore the previous graphics port (S
etPort
, using the value from step 6).End the current page (
PMSessionEndPage
).Once steps 5 through 12 have been performed for all the requested pages, signal the completion of the print job (
PMSessionEndDocument
).
Handling Errors
You need to write code that handles all the errors that might occur during printing. Part of this support includes a procedure to display alerts with their messages in the human language that most closely matches the user's language preferences. See Internationalization for details on how to do this properly.
Save as PDF
Because Mac OS X stores document pages as PDF files during the spooling process, it is very easy to add support for saving a document as a PDF. You can do this as follows:
Direct your printing code to print to a file instead of a printer.
Add code that gets the desired filename and file location from the user.
Decide whether or not the Page Setup and Print dialogs should be part of the "save as PDF" process. If you decide they should not be (the usual case), you must add code that automatically adds reasonable page-related values to substitute for those normally provided by the Page Setup and Print dialogs.
Start the printing process.
By adding these changes, you can use the same code to print a document or save it as a PDF file.
For Further Information
The links below point to the documentation you will need to get started with implementing printing using the Carbon Printing Manager.
Mac OS X Printing page | |
Mac OS X Printing Documentation page | |
the Carbon Printing Manager documentation page | Carbon Printing Manager Reference |
About the Mac OS X Printing System | Mac OS X Printing System Overview |
Extending Printing Dialogs | |
Printing Plug-In Interfaces Reference | Printing Plug-in Interfaces Reference |
Carbon Printing Manager header files |
|
Copyright © 2003, 2011 Apple Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2011-01-05